Common Mistakes and Solutions when Using the charAt Method in Java’s String Class
2023-12-06 | by reerr.com
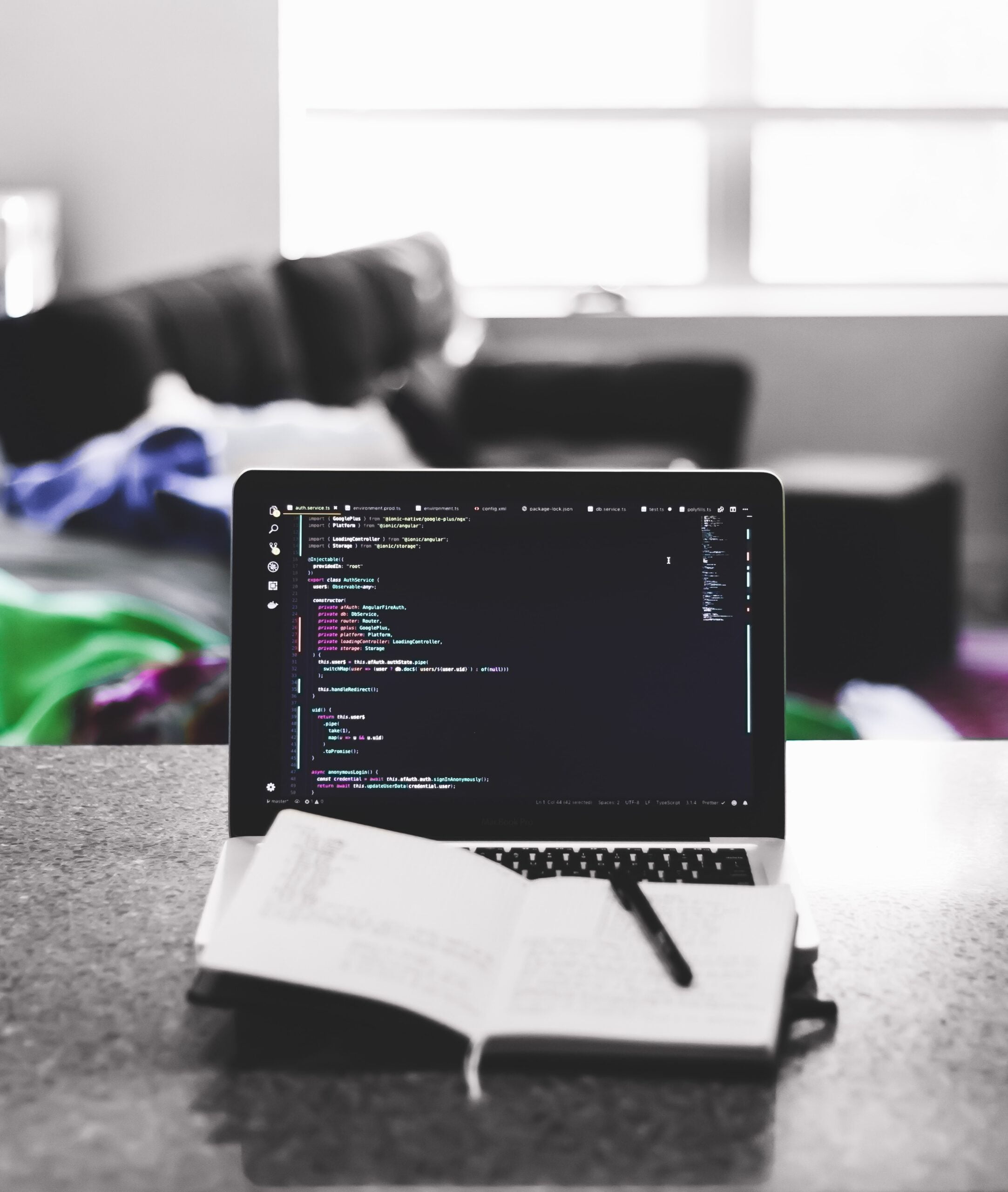
The charAt
method in Java’s String class is a useful tool for retrieving a specific character from a string based on its position. However, there are some common mistakes that developers may encounter when using this method. In this article, we will explore these mistakes and provide solutions to avoid them.
Common Mistake: IndexOutOfBoundsException
Cause: One common mistake is encountering an IndexOutOfBoundsException
when using the charAt
method. This exception is thrown when the index provided is outside the range of the string’s length.
Solution: To avoid this error, it is essential to check the length of the string before calling the charAt
method. This can be done by using an if
statement to ensure that the index is within the string’s length.
String str = "Hello, world!";
int index = 4;
char ch;
if(index >= 0 && index < str.length()) {
ch = str.charAt(index);
}
In the example above, we first declare a string variable str
and assign it the value “Hello, world!”. We then declare an integer variable index
and assign it the value 4, representing the desired position of the character we want to retrieve. Finally, we use an if
statement to check if the index is within the valid range before calling the charAt
method.
Common Mistake: Incorrect Indexing
Cause: Another mistake that can occur is using incorrect indexing when calling the charAt
method. It is important to remember that indexing in Java starts at 0, so the first character of a string is at index 0.
Solution: To avoid this mistake, make sure to adjust the index accordingly. For example, if you want to retrieve the fifth character of a string, you should use an index of 4.
String str = "Hello, world!";
char ch = str.charAt(4); // Returns 'o'
In the example above, str.charAt(4)
returns the fifth character (‘o’) of the string “Hello, world!”.
Conclusion
The charAt
method in Java’s String class is a powerful tool for retrieving specific characters from a string. By avoiding common mistakes such as IndexOutOfBoundsException
and incorrect indexing, developers can effectively utilize this method in their code. Remember to always check the length of the string and adjust the index accordingly to ensure the correct usage of the charAt
method.
By understanding these common mistakes and their solutions, developers can enhance their proficiency in using the charAt
method and produce more reliable and error-free code.
RELATED POSTS
View all