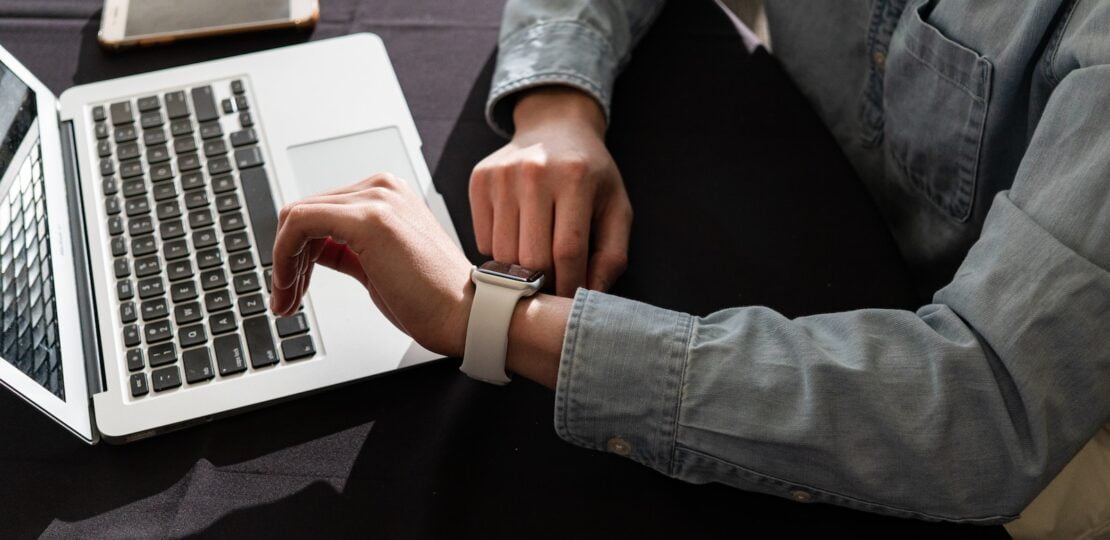
Introduction to LocalDateTime
In Java 8 and later versions, the LocalDateTime
class is part of the Date and Time API. It is used to represent date and time without any timezone information. This class provides various methods to handle date and time together, making it a powerful tool for developers.
Basic Usage of LocalDateTime
Let’s explore some basic ways to use LocalDateTime
:
- Get Current Date and Time
- Set a Specific Date and Time
- Format Date and Time
- Parse Date and Time
- Manipulate Date and Time
- Compare Dates and Times
LocalDateTime now = LocalDateTime.now();
This code snippet creates a LocalDateTime
object representing the current date and time.
LocalDateTime specificDateTime = LocalDateTime.of(2023, Month.JANUARY, 29, 20, 45, 55);
Here, we create a LocalDateTime
object for a specific date and time: January 29, 2023, at 8:45:55 PM.
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
String formattedDateTime = now.format(formatter);
Using a DateTimeFormatter
, we can format the LocalDateTime
object into a specific pattern. In this example, the date and time are formatted as “yyyy-MM-dd HH:mm:ss”.
LocalDateTime parsedDateTime = LocalDateTime.parse("2023-01-29T20:45:55");
We can create a LocalDateTime
object from a string representation of a date and time. The string “2023-01-29T20:45:55” is parsed into a LocalDateTime
object.
LocalDateTime tomorrow = now.plusDays(1);
LocalDateTime nextHour = now.plusHours(1);
Since LocalDateTime
is immutable, any manipulation of date and time creates a new instance. In the above examples, we add one day and one hour to the current date and time to get the next day and the next hour, respectively.
boolean isBefore = now.isBefore(specificDateTime);
boolean isAfter = now.isAfter(specificDateTime);
boolean isEqual = now.isEqual(specificDateTime);
We can compare LocalDateTime
instances to check if a date and time is before, after, or equal to another. The above code snippet demonstrates how to perform such comparisons.
Considerations for Timezone Information
It is important to note that LocalDateTime
does not contain any timezone information. If your application requires global compatibility and accurate timezone handling, consider using ZonedDateTime
or OffsetDateTime
instead.
ZonedDateTime
represents a date and time with timezone information, allowing you to work with different time zones. On the other hand, OffsetDateTime
represents a date and time with an offset from UTC (Coordinated Universal Time).
By utilizing ZonedDateTime
or OffsetDateTime
when necessary, you can ensure accurate and reliable handling of timezones in your Java applications.
Conclusion
In this blog post, we explored the basic usage of the LocalDateTime
class in Java 8 and later versions. We learned how to get the current date and time, set specific date and time, format and parse date and time, manipulate date and time, and compare different instances of LocalDateTime
.
While LocalDateTime
is a powerful class for handling date and time, it lacks timezone information. For applications that require timezone awareness, it is recommended to use ZonedDateTime
or OffsetDateTime
to ensure accurate and reliable time handling.
RELATED POSTS
View all