Understanding the Differences Between Null and Undefined in JavaScript
2023-11-20 | by reerr.com
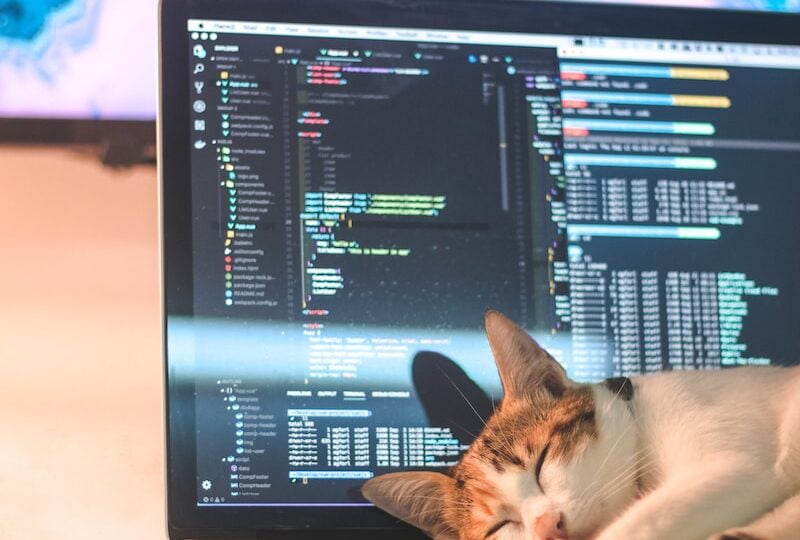
In JavaScript, both null and undefined represent “no value.” However, there are a few differences between them:
- Declaration: null is a value that is explicitly assigned to represent ‘no value.’ On the other hand, undefined refers to a variable that has not yet been assigned a value.
let testVar; // undefined
let testVar = null; // null
- typeof operator: typeof null returns “object” and typeof undefined returns “undefined”. This is considered a bug in JavaScript as null is not actually an object.
console.log(typeof null); // "object"
console.log(typeof undefined); // "undefined"
- Comparison: The two values behave differently when compared using the strict equality (===) and the loose equality (==) operators. null is only equal to undefined when using the loose equality operator.
console.log(null === undefined); // false
console.log(null == undefined); // true
- Default values in functions: When setting default parameter values in functions, if the parameter is passed undefined, the default value is used. However, if null is passed, null is used.
function re(val = 'default') {
console.log(val);
}
re(undefined); // prints 'default'
re(null); // prints 'null'
Because of these differences, null and undefined are handled differently in your programs. Typically, undefined is used to represent uninitialized variables, functions without a return value, or missing object properties. In contrast, null is used by programmers to represent a deliberately empty or unknown value.
Unlike JavaScript, in Java, only null exists and there is no undefined.
RELATED POSTS
View all