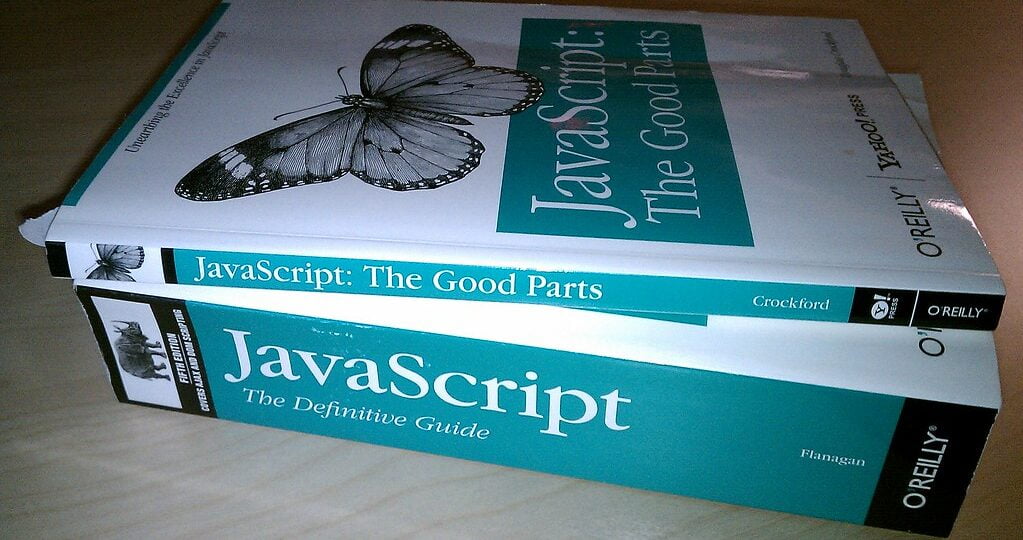
The Document Object Model (DOM) is an essential part of HTML document structure, but beginners often have misconceptions about its role and how to manipulate it. Understanding the correct usage of DOM manipulation methods and the characteristics of the JavaScript interpreter is crucial for efficient JavaScript coding. Let’s explore some common misunderstandings and provide examples to clarify these concepts.
DOM Manipulation
DOM manipulation involves accessing and modifying HTML elements using JavaScript. Two commonly used methods for accessing elements are document.getElementById
and document.querySelector
. However, beginners often overlook the fact that these methods can only be used after the DOM is fully loaded.
For example, consider the following code:
// Ensure the DOM is fully loaded before accessing HTML elements
document.addEventListener('DOMContentLoaded', function() {
let myElement = document.getElementById('myElement');
myElement.style.color = 'red';
});
In this code, the DOMContentLoaded
event listener ensures that the DOM is fully loaded before accessing and manipulating the myElement
. This approach prevents errors that may occur if the element hasn’t been loaded onto the page yet.
JavaScript Interpreter Characteristics
The JavaScript interpreter parses and executes code line by line. Beginners often misunderstand characteristics like hoisting and scope, which can lead to unexpected behavior in their code.
Consider the following example:
console.log(myVar); // undefined
var myVar = 10;
console.log(myVar); // 10
In this example, the variable myVar
is declared using the var
keyword. However, due to hoisting, the declaration of myVar
is moved to the top of the code. As a result, the first console.log
outputs undefined
. Understanding hoisting is crucial to avoid confusion about the execution results of the code.
Correctly understanding DOM manipulation and the features of the JavaScript interpreter is essential for efficient JavaScript coding. Beginners should take the time to familiarize themselves with these basic concepts to minimize mistakes in JavaScript development.
RELATED POSTS
View all