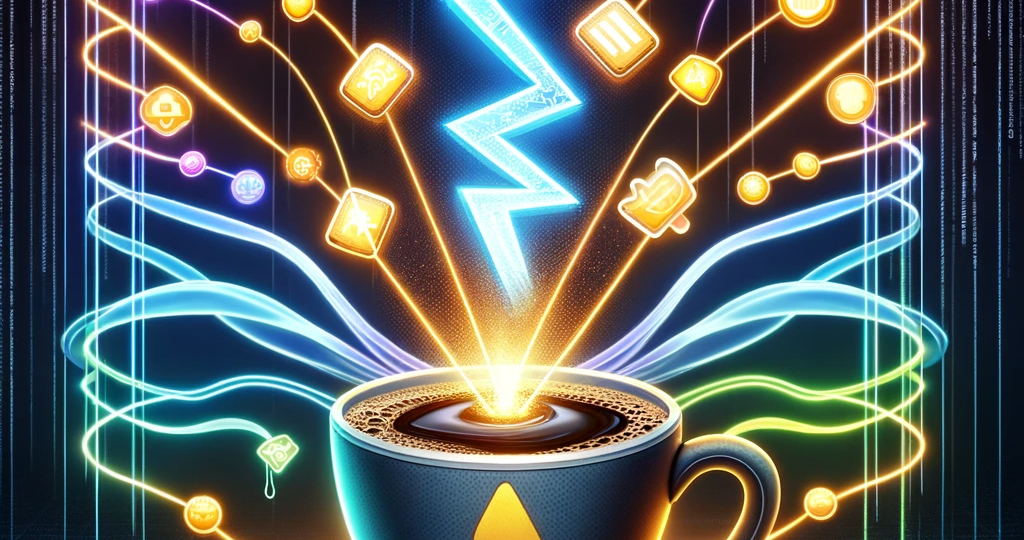
Thread interruption is a crucial aspect of managing multithreaded applications in Java. It allows us to gracefully stop or alter the behavior of a thread, ensuring efficient and controlled execution. In this blog post, we will explore the concept of thread interruption, the role of Thread.interrupt(), when to use it, how to implement it effectively, common mistakes to avoid, and advanced tips for Java concurrency.
What is Thread.interrupt()?
Thread.interrupt() is a method in Java that plays a significant role in signaling to a thread that it should stop its current operations or alter its behavior. When called on a thread, it sets the thread’s interruption status to true, indicating that it has been interrupted. However, it does not forcefully stop the thread; it is up to the thread itself to handle the interruption and decide how to respond.
When to Use Thread.interrupt()
There are various scenarios where using Thread.interrupt() is appropriate. One common use case is when you need to stop a thread gracefully. Instead of abruptly terminating the thread, which can lead to resource leaks or inconsistent application state, you can interrupt the thread and let it handle the interruption in a controlled manner. Thread.interrupt() is also useful when you want to handle thread termination in a specific way, such as cleaning up resources or notifying other threads.
How to Implement Thread.interrupt() Effectively
Checking Interruption Status
Threads can check their interruption status using two methods: Thread.interrupted() and isInterrupted(). Thread.interrupted() is a static method that checks the interruption status of the current thread and clears it. On the other hand, isInterrupted() is an instance method that checks the interruption status of a specific thread without clearing it. It is essential to understand the difference between these methods to handle thread interruption correctly.
Handling InterruptedException
When a thread is interrupted, it may encounter an InterruptedException while executing certain blocking operations such as sleep() or wait(). It is crucial to properly handle InterruptedException to maintain thread responsiveness. Ignoring or mishandling this exception can lead to unresponsive threads or unexpected behavior. The general pattern for handling InterruptedException is to catch the exception, perform any necessary cleanup or state management, and then either rethrow the exception or exit the thread gracefully.
Examples and Code Snippets
Let’s take a look at some practical examples and code snippets that demonstrate the correct implementation of Thread.interrupt() in various scenarios:
// Example 1: Gracefully stopping a thread
public class MyThread extends Thread {
private volatile boolean running = true;
public void run() {
while (running) {
// Perform some task
if (Thread.interrupted()) {
running = false;
}
}
}
public void stopThread() {
running = false;
interrupt();
}
}
// Example 2: Handling InterruptedException
public void run() {
try {
while (!Thread.interrupted()) {
// Perform some blocking operation
}
} catch (InterruptedException e) {
// Properly handle the exception
Thread.currentThread().interrupt();
}
}
Common Mistakes and How to Avoid Them
When using Thread.interrupt(), there are some common mistakes that developers should be aware of:
- Forgetting to handle InterruptedException properly, leading to unresponsive threads or unexpected behavior.
- Assuming that Thread.interrupt() immediately stops the thread, when in reality, it is a cooperative mechanism.
- Not checking the interruption status regularly within the thread’s execution loop.
To avoid these pitfalls, always handle InterruptedException, understand the cooperative nature of Thread.interrupt(), and regularly check the interruption status within the thread.
Advanced Tips for Java Concurrency
For developers looking to dive deeper into Java concurrency, understanding Thread.interrupt() is just the beginning. Advanced topics include thread pools, executor services, and futures. These concepts provide higher-level abstractions for managing threads and can greatly simplify concurrent programming. When using these advanced techniques, it is still essential to correctly utilize Thread.interrupt() to ensure proper thread management and responsiveness.
Conclusion
Thread interruption is a powerful mechanism in Java for managing multithreaded applications. By using Thread.interrupt() effectively, developers can gracefully stop threads, handle thread termination, and maintain thread responsiveness. It is crucial to understand the proper usage of Thread.interrupt(), handle InterruptedException correctly, and be aware of common mistakes to avoid. As you explore more advanced topics in Java concurrency, remember to incorporate Thread.interrupt() into your code and practice these techniques to create efficient and responsive multithreaded applications.
RELATED POSTS
View all