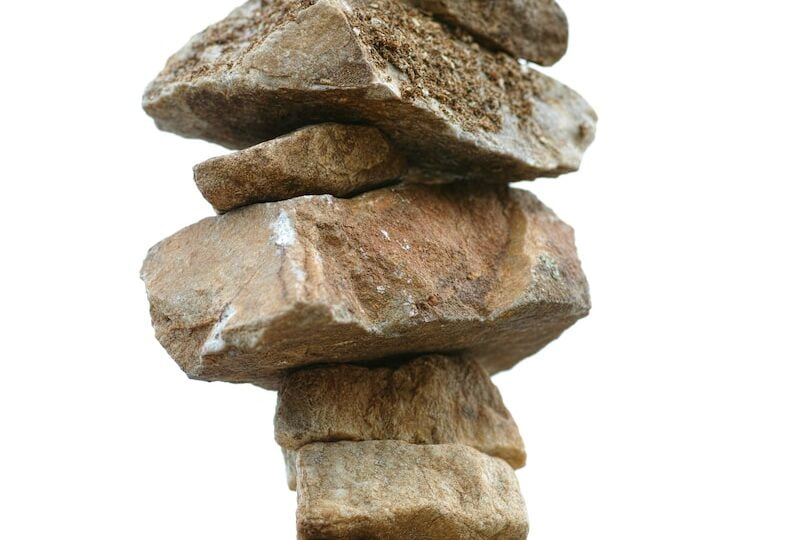
Introduction
BufferedReader in Java is a critical class used for reading text from an input stream. It’s efficient and popular, but there are common pitfalls that many developers fall into. In this post, we’ll explore the top seven mistakes to avoid when using BufferedReader and provide tips to enhance your Java programming skills.
1. Not Closing the BufferedReader
A major pitfall in using BufferedReader in Java is failing to close the stream. When you forget to close your BufferedReader, it can lead to resource leaks and occupy memory unnecessarily. Always use a try-with-resources statement or close it in a finally block.
2. Ignoring Exception Handling
Handling exceptions is crucial to avoid BufferedReader pitfalls. Not handling IOExceptions can crash your program unexpectedly. Always include proper exception handling to ensure your code is robust and error-resistant.
3. Improper Buffer Size
Choosing the wrong buffer size is a common BufferedReader mistake. Using a very small or large buffer size can affect performance. Understand the nature of your data and environment to choose an appropriate buffer size.
4. Misunderstanding ReadLine
A common error in Java BufferedReader usage involves misunderstanding the readLine method. Remember that readLine() returns null at the end of the stream. Ensure your code checks for this condition to avoid NullPointerExceptions.
5. Inefficient Reading Patterns
Avoid inefficient reading patterns to prevent BufferedReader pitfalls. Repeatedly calling read() for single characters is inefficient. Use readLine() for reading lines, or read(char[] cbuf) for bulk reading.
6. Ignoring Character Encoding
Overlooking character encoding is a frequent Java BufferedReader error. Always specify the appropriate character encoding when reading text data. Ignoring this can lead to incorrect data interpretation, especially with non-ASCII characters.
7. Concurrent Access Issues
Concurrent access is a tricky aspect of using BufferedReader in Java. BufferedReader is not thread-safe. If you need concurrent access, synchronize it externally or use alternative thread-safe classes.
By avoiding these common mistakes, you can enhance your coding efficiency and accuracy when using BufferedReader in Java. Keep these tips in mind to improve your Java programming skills and write more robust code.
RELATED POSTS
View all