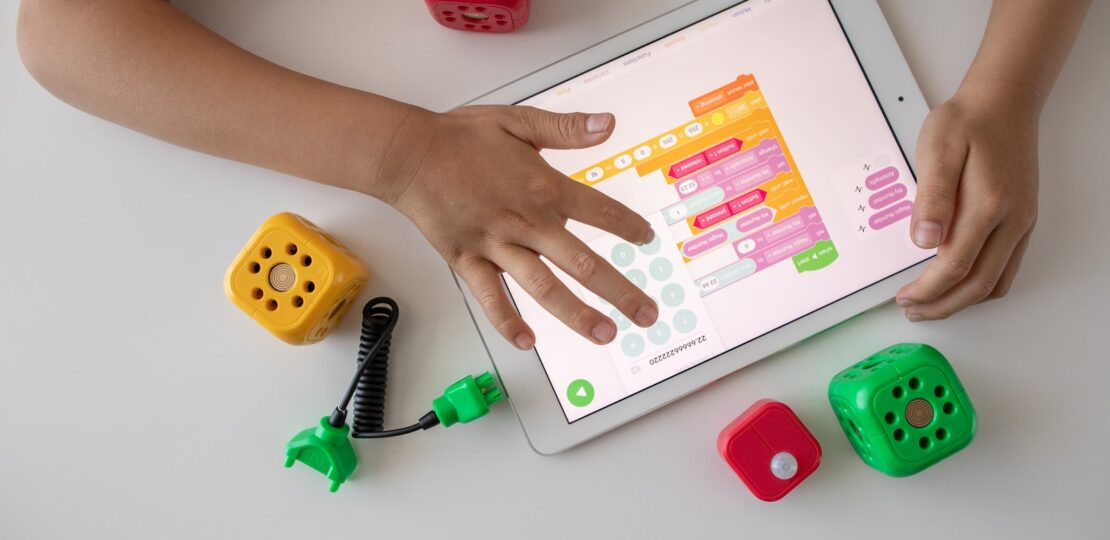
Introduction
A for loop is a common control flow statement in programming languages that allows you to repeatedly execute a block of code based on a specified condition. It is often used when you have a known number of iterations or when you want to iterate over a collection of elements. In this article, we will explore examples of for loops in different programming languages.
1. Python
# Syntax:
# for variable in sequence:
# code to be executed
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
In Python, you can use a for loop to iterate over a sequence, such as a list, tuple, or string. In the example above, the for loop iterates over the “fruits” list and prints each fruit on a new line.
2. JavaScript
// Syntax:
// for (initialization; condition; increment/decrement) {
// code to be executed
// }
var fruits = ["apple", "banana", "cherry"];
for (var i = 0; i < fruits.length; i++) {
console.log(fruits[i]);
}
In JavaScript, you can use a for loop with three parts: initialization, condition, and increment/decrement. The loop starts with the initialization, then checks the condition before each iteration, and increments the counter after each iteration. The example above demonstrates how to iterate over the “fruits” array and log each fruit to the console.
3. Java
// Syntax:
// for (initialization; condition; increment/decrement) {
// code to be executed
// }
String[] fruits = {"apple", "banana", "cherry"};
for (int i = 0; i < fruits.length; i++) {
System.out.println(fruits[i]);
}
In Java, the syntax for a for loop is similar to JavaScript. The example above shows how to iterate over the “fruits” array and print each fruit using the System.out.println() method.
4. C
// Syntax:
// for (initialization; condition; increment/decrement) {
// code to be executed
// }
#include
int main() {
char fruits[3][10] = {"apple", "banana", "cherry"};
for (int i = 0; i < 3; i++) {
printf("%sn", fruits[i]);
}
return 0;
}
In C, you can use a for loop to iterate over an array. The example above demonstrates how to iterate over the “fruits” array and print each fruit using the printf() function.
5. Ruby
# Syntax:
# for variable in collection do
# code to be executed
# end
fruits = ["apple", "banana", "cherry"]
for fruit in fruits do
puts fruit
end
In Ruby, you can use a for loop to iterate over a collection, such as an array. The example above shows how to iterate over the “fruits” array and output each fruit using the puts method.
Conclusion
For loops are a fundamental construct in programming languages that allow you to iterate over a sequence of elements. Each programming language has its own syntax for implementing for loops, but they all serve the same purpose of executing a block of code repeatedly. By understanding how for loops work in different languages, you can become a more versatile programmer and apply this knowledge to solve various problems.
RELATED POSTS
View all