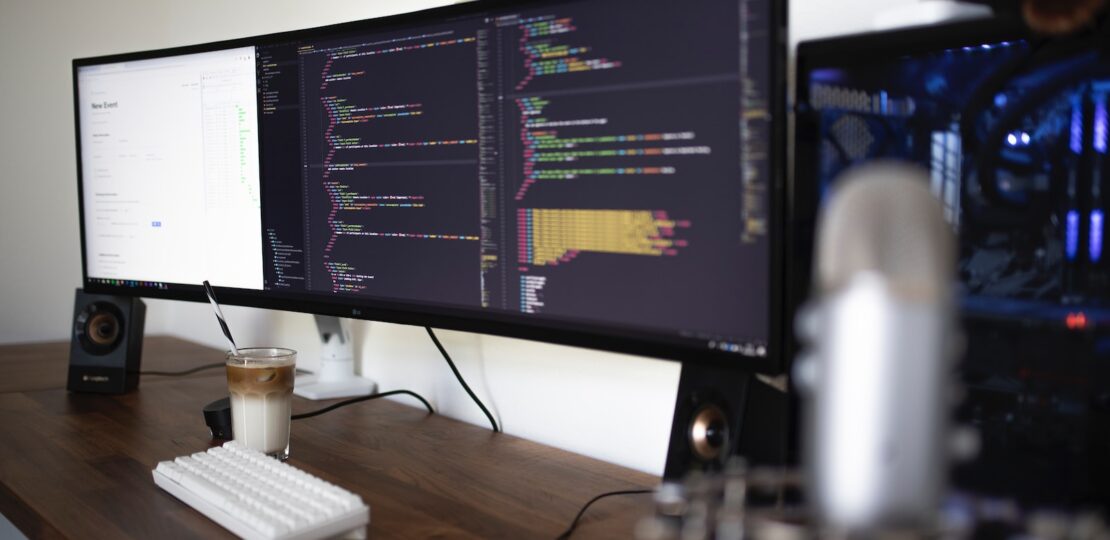
In Java, the Repository interface plays a crucial role in the data access layer, commonly used in frameworks like Spring Data JPA, MyBatis, or other ORM (Object-Relational Mapping) frameworks. It abstracts the database operations, making it easier to interact with the data layer.
In Spring Data JPA, interfaces like CrudRepository or JpaRepository provide a range of useful methods. These methods are fundamental for querying, saving, updating, and deleting data. Let’s take a closer look at some of the key methods:
- save(S entity): Saves the given entity and returns the saved entity. It updates the entity if it already exists.
- findById(ID id): Finds the entity by its ID and returns it wrapped in an Optional.
- findAll(): Returns all entities in a list.
- count(): Returns the total number of entities in the repository.
- delete(S entity): Deletes the given entity.
- deleteById(ID id): Deletes the entity with the given ID.
- existsById(ID id): Checks whether an entity with the given ID exists.
These methods provide a convenient way to perform common database operations without having to write custom SQL queries. They are especially useful when working with large datasets or complex database structures.
JpaRepository extends CrudRepository and offers additional features specific to JPA, like methods for handling pagination and sorting. This makes it easier to retrieve data in smaller chunks and order the results based on specific criteria. For example, you can use methods like findAll(Pageable pageable) to retrieve data in paginated form or findAll(Sort sort) to retrieve data sorted by a specific field.
In addition to these methods, Spring Data JPA also offers the capability to automatically create queries based on method names. This is known as Query Creation from Method Names. By following a specific naming convention, you can define custom queries without writing any SQL code.
For example, declaring a method like findByUsername(String username)
in the repository interface allows Spring Data JPA to automatically generate a query to find entities by the username. This feature saves developers from writing repetitive boilerplate code for common query scenarios.
It’s important to note that while Spring Data JPA provides a wide range of pre-defined methods, there may be cases where you need to write custom queries using the @Query annotation. This allows you to write complex queries in SQL or JPQL (Java Persistence Query Language) when the built-in methods are not sufficient.
In conclusion, the Repository interface in Java, particularly in frameworks like Spring Data JPA, simplifies the interaction with the data access layer. It abstracts the database operations and provides a set of convenient methods for querying, saving, updating, and deleting data. By leveraging the power of the Repository interface, developers can focus more on the business logic and spend less time dealing with low-level database operations.
RELATED POSTS
View all