Mitigating SQL Injection Attacks in Java: Filtering Special Characters
2023-11-24 | by reerr.com
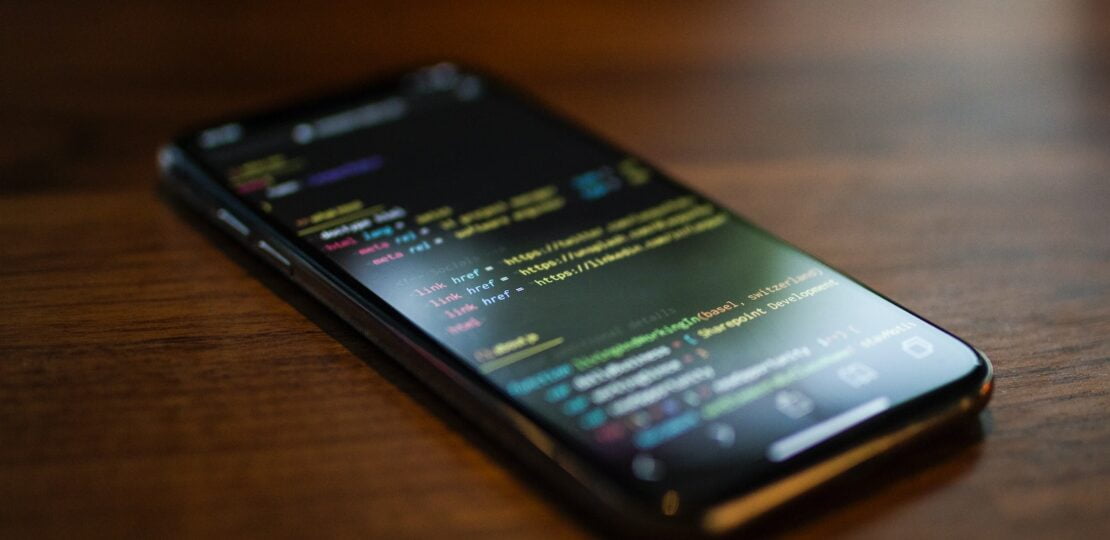
Mitigating SQL Injection Attacks in Java: Filtering Special Characters
When it comes to application development, security should always be the top priority, particularly when dealing with databases. One of the most serious cybersecurity risks that developers face is SQL Injection attacks, where hackers attempt to manipulate SQL queries through user inputs. Fortunately, there are coding techniques that can help mitigate this risk, such as filtering special characters. In this example, we will demonstrate how to implement this approach in Java.
Understanding SQL Injection Attacks
Before diving into the solution, it’s important to understand what SQL Injection attacks are and how they work. In a nutshell, SQL Injection is a type of attack where an attacker inserts malicious SQL code into a query, which can then be executed by the database. This allows the attacker to manipulate the query and potentially gain unauthorized access to sensitive data or even modify the database itself.
The Importance of Filtering Special Characters
One effective way to prevent SQL Injection attacks is by filtering special characters from user inputs. Special characters, such as single quotes (‘), double quotes (“), and semicolons (;), can be used by attackers to break out of the intended SQL query and inject their own malicious code. By filtering these characters, we can ensure that user inputs are treated as literal values and not as part of the SQL query.
Implementing Special Character Filtering in Java
In Java, there are several ways to implement special character filtering. One common approach is to use prepared statements or parameterized queries, which automatically handle the escaping of special characters. Here’s an example:
String username = request.getParameter("username");
String password = request.getParameter("password");
String sql = "SELECT * FROM users WHERE username = ? AND password = ?";
PreparedStatement statement = connection.prepareStatement(sql);
statement.setString(1, username);
statement.setString(2, password);
ResultSet resultSet = statement.executeQuery();
In this example, we retrieve the username and password inputs from the user. Instead of directly concatenating them into the SQL query, we use placeholders (?) and set the values using the setString()
method of the PreparedStatement
class. This ensures that the inputs are properly escaped and treated as literal values, preventing any potential SQL Injection attacks.
Another approach is to use input validation and sanitization techniques to filter out special characters. This can be done using regular expressions or custom validation methods. Here’s an example:
String username = request.getParameter("username");
String password = request.getParameter("password");
if (!username.matches("[a-zA-Z0-9]+") || !password.matches("[a-zA-Z0-9]+")) {
// Handle invalid inputs
} else {
// Proceed with the SQL query
}
In this example, we use regular expressions to ensure that the username and password inputs only contain alphanumeric characters. If any special characters are detected, we can handle them accordingly, such as displaying an error message or rejecting the input altogether.
Conclusion
SQL Injection attacks pose a significant threat to the security of applications that interact with databases. By implementing proper coding techniques, such as filtering special characters, developers can greatly reduce the risk of these attacks. In this example, we demonstrated how to perform special character filtering in Java using prepared statements and input validation. Remember, security should always be a top priority in application development, and taking proactive measures to prevent SQL Injection attacks is essential.
RELATED POSTS
View all