Understanding LinkedLists: A Fundamental Data Structure Explained
2023-12-15 | by reerr.com
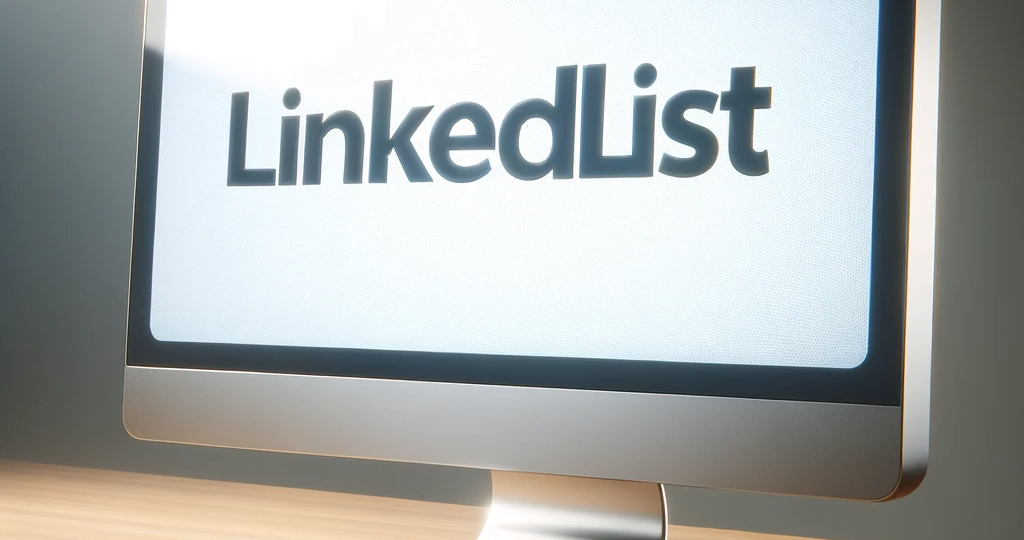
LinkedList Explained
A LinkedList is a fundamental data structure in computer science and programming. It consists of a sequence of nodes, where each node contains a data value and a reference (or link) to the next node in the sequence. This structure allows for efficient insertion and deletion of elements as it doesn’t require reorganizing the entire data structure, unlike an array.
Key Characteristics
- Dynamic Size: Unlike arrays, LinkedLists are dynamic and can grow or shrink in size.
- Sequential Access: Elements are accessed sequentially, starting from the head of the list.
- Memory Utilization: Efficient memory usage since it allocates memory as needed.
Common Mistakes When Using LinkedLists
While LinkedLists offer many advantages, there are some common mistakes that programmers should be aware of:
- Forgetting to Update Pointers: When adding or removing nodes, it’s crucial to correctly update the pointers of adjacent nodes. Failing to do so can break the list.
- Not Handling the Head and Tail Correctly: Special care is needed when inserting or deleting nodes at the beginning or end of the list. For instance, adding a new head requires updating the head pointer.
- Memory Leaks: In languages like C, not properly deallocating the memory of a deleted node can lead to memory leaks.
- Inefficient Operations: Misusing LinkedLists for operations that require frequent random access can be inefficient due to its sequential nature.
- Infinite Loops: When traversing a LinkedList, if the end condition is not correctly defined, it can lead to an infinite loop, especially in circular LinkedLists.
- Null Pointer Exceptions: Accessing nodes without checking if they are null can cause exceptions, particularly in languages like Java.
- Concurrent Modifications: Modifying a LinkedList while it’s being iterated over can cause unpredictable behavior, especially in multithreaded environments.
Conclusion
Understanding and correctly implementing LinkedLists can significantly enhance a programmer’s ability to manage data efficiently. Being mindful of these common mistakes will help in writing more robust and error-free code. Remember, practice and careful attention to detail are key in mastering the use of LinkedLists.
RELATED POSTS
View all