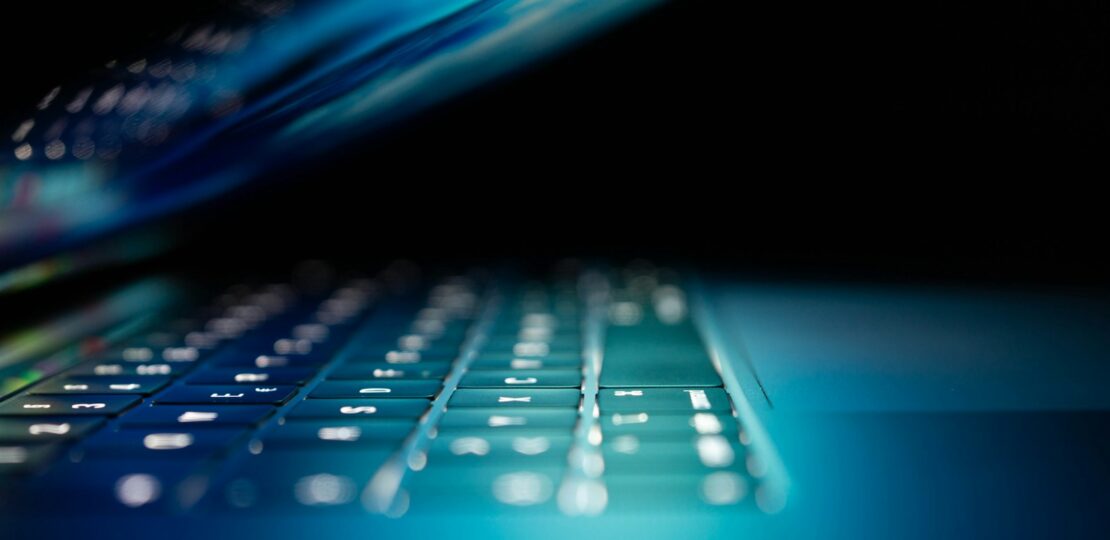
Introduction
Application security is of utmost importance in today’s digital landscape, and one of the most common and dangerous security threats is the SQL injection attack. This type of attack allows hackers to manipulate SQL queries to perform unauthorized actions on your system. To safeguard your application from such attacks, it is crucial to follow safe coding practices. In this blog post, we will explore how to protect your Python application from SQL injection attacks.
The Dangers of Unsafe Coding
One common mistake that developers make is directly including user input in SQL queries. This practice is highly unsafe and can easily expose your application to SQL injection attacks. Let’s take a look at an example:
import sqlite3
def get_user_data(username):
conn = sqlite3.connect("database.db")
cursor = conn.cursor()
query = "SELECT * FROM users WHERE username = '" + username + "'"
cursor.execute(query)
data = cursor.fetchall()
conn.close()
return data
In the above code snippet, the get_user_data
function takes a username
parameter and directly includes it in the SQL query. This approach is vulnerable to SQL injection attacks because an attacker can manipulate the username
input to inject malicious SQL code.
Safe Coding Practices
To protect your application from SQL injection attacks, you should always use parameterized queries or prepared statements. These techniques allow you to separate the SQL code from the user input, making it impossible for attackers to inject malicious code.
Here’s an example of how to use parameterized queries in Python:
import sqlite3
def get_user_data(username):
conn = sqlite3.connect("database.db")
cursor = conn.cursor()
query = "SELECT * FROM users WHERE username = ?"
cursor.execute(query, (username,))
data = cursor.fetchall()
conn.close()
return data
In the modified code snippet, we use a question mark (?)
as a placeholder for the user input. The actual value of username
is passed separately as a parameter to the execute
method. This ensures that the user input is treated as data and not as part of the SQL code, effectively preventing SQL injection attacks.
Additional Security Measures
While using parameterized queries is a crucial step in protecting your application from SQL injection attacks, it is also important to implement other security measures. Some additional best practices include:
- Implementing input validation to ensure that user input follows the expected format and type.
- Applying proper access controls and user authentication to restrict unauthorized access to sensitive data.
- Regularly updating and patching your application and database software to address any security vulnerabilities.
- Conducting regular security audits and penetration testing to identify and fix any potential vulnerabilities.
Conclusion
SQL injection attacks can have severe consequences for your application’s security and integrity. By following safe coding practices, such as using parameterized queries, you can effectively protect your Python application from these attacks. Remember to also implement additional security measures and stay updated with the latest security best practices to ensure the overall security of your application.
RELATED POSTS
View all